Research and Innovation
- Consensus algorithm research
- Blockchain expansion
- Privacy
- Reduce digital currency carbon emissions
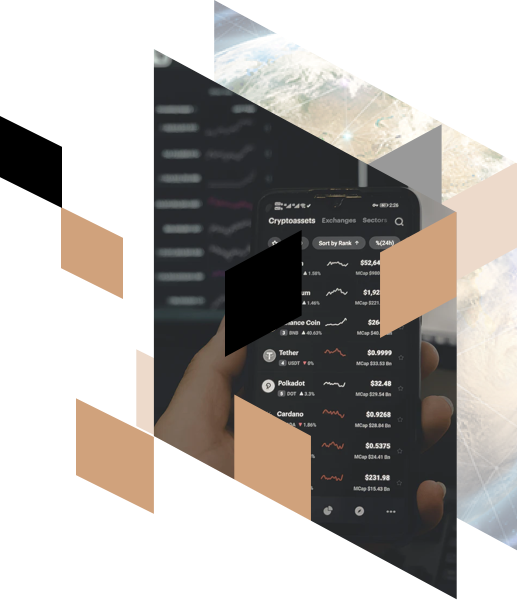
ORACLES

PREREQUISITES
Make sure you’re familiar with nodes, consensus mechanisms, and smart contract anatomy, specifically events.WHAT IS AN ORACLE
An oracle is a bridge between the blockchain and the real world. They act as on-chain APIs you can query to get information into your smart contracts. This could be anything from price information to weather reports. Oracles can also be bi-directional, used to “send” data out to the real world.WHY ARE THEY NEEDED?
With a blockchain like Kinglory you need every node in the network to be able to replay every transaction and end up with the same result, guaranteed. APIs introduce potentially variable data. If you were sending someone an amount of KGC based on an agreed $USD value using a price API, the query would return a different result from one day to the next. Not to mention, the API could be hacked or deprecated. If this happens, the nodes in the network wouldn’t be able to agree on Kinglory’s current state, effectively breaking consensus.
Oracles solve this problem by posting the data on the blockchain. So any node replaying the transaction will use the same immutable data that’s posted for all to see. To do this, an oracle is typically made up of a smart contract and some off-chain components that can query APIs, then periodically send transactions to update the smart contract’s data.
- Security
- Architecture
This is an example of a simple Oracle architecture, but there are more ways than this to trigger off-chain computation.
- Emit a log with your smart contract event.
- An off-chain service has subscribed (usually using something like the JSON-RPC KGC_subscribe command) to these specific logs.
- The off-chain service proceeds to do some tasks as defined by the log.
The off-chain service responds with the data requested in a secondary transaction to the smart contract.
This is how to get data in a 1 to 1 manner, however to improve security you may want to decentralize how you collect your off-chain data.
The next step might be to have a network of these nodes making these calls to different APIs and sources, and aggregating the data on-chain.
Chainlink Off-Chain Reporting (Chainlink OCR) has improved on this methodology by having the off-chain oracle network communicate with each other, cryptographically sign their responses, aggregate their responses off-chain, and send only 1 transaction on-chain with the result. This way, fewer gas is spent but you still get the guarantee of decentralized data since every node has signed their part of the transaction, making it unchangeable by the node sending the transaction. If the node doesn’t transact, the escalation policy kicks in, and the next node sends the transaction.
USAGE

Using services like Chainlink, you can reference decentralized data on-chain, that has already been pulled from the real world and aggregated. Sort of like a public commons, but for decentralized data. You can also build your own modular oracle networks to get any customized data you’re looking for. In addition, you can do off-chain computation and send information to the real world as well. Chainlink has infrastructure in place to:
- Get crypto price feeds in your contract
- Generate verifiable random numbers (useful for gaming)
- Call external APIs – one novel use of this is Checking wBTC reserves
This is an example of how to get the latest KGC price in your smart contract using a Chainlink price feed:
• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink Data Feeds

Chainlink Data Feeds

• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink VRF (Verifiable Random Function) is a provably-fair and verifiable source of randomness designed for smart contracts. Smart contract developers can use Chainlink VRF as a tamper-proof random number generation (RNG) to build reliable smart contracts for any applications which rely on unpredictable outcomes:
- Blockchain games and NFTs
- Random assignment of duties and resources (e.g. randomly assigning judges to cases)
- Choosing a representative sample for consensus mechanisms
Random numbers are difficult because blockchains are deterministic.
Working with Chainlink Oracles outside of data feeds follows the request and recieve cycle of working with Chainlink. They use the LINK token to send oracle providers oracle gas for returning responses. The LINK token is specifically designed to work with oracles and are based on the upgraded ERC-677 token, which is backwards compatible with ERC-20. The following code, if deployed on the Kovan testnet will retreive a cryptographically proven random number. To make the request, fund the contract with some testnet LINK token that you can get from the Kovan LINK Faucet.
• 1 • 2pragma solidity 0.6.6; • 3 • 4import "@chainlink/contracts/src/v0.6/VRFConsumerBase.sol"; • 5 • 6contract RandomNumberConsumer is VRFConsumerBase { • 7 • 8 bytes32 internal keyHash; • 9 uint256 internal fee; • 10 • 11 uint256 public randomResult; • 12 • 13 /** • 14 * Constructor inherits VRFConsumerBase • 15 * • 16 * Network: Kovan • 17 * Chainlink VRF Coordinator address: 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9 • 18 * LINK token address: 0xa36085F69e2889c224210F603D836748e7dC0088 • 19 * Key Hash: 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4 • 20 */ • 21 constructor() • 22 VRFConsumerBase( • 23 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9, // VRF Coordinator • 24 0xa36085F69e2889c224210F603D836748e7dC0088 // LINK Token • 25 ) public • 26 { • 27 keyHash = 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4; • 28 fee = 0.1 * 10 ** 18; // 0.1 LINK (varies by network) • 29 } • 30 • 31 /** • 32 * Requests randomness from a user-provided seed • 33 */ • 34 function getRandomNumber(uint256 userProvidedSeed) public returns (bytes32 requestId) { • 35 require(LINK.balanceOf(address(this)) >= fee, "Not enough LINK - fill contract with faucet"); • 36 return requestRandomness(keyHash, fee, userProvidedSeed); • 37 } • 38 • 39 /** • 40 * Callback function used by VRF Coordinator • 41 */ • 42 function fulfillRandomness(bytes32 requestId, uint256 randomness) internal override { • 43 randomResult = randomness; • 44 } • 45}
Chainlink VRF
Chainlink API Call

Chainlink API Calls are the easiest way to get data from the off-chain world in the traditional way the web works: API calls. Doing a single instance of this and having only 1 oracle makes it centralized by nature. In order to keep it truly decentralized a smart contract platform would need to use numerous nodes, found in an external data market.
Deploy the following code in remix on the kovan network to test.
This also follows the request and receive cycle of oracles, and needs the contract to be funded with Kovan LINK (the oracle gas) in order to work.
• 1pragma solidity ^0.6.0; • 2 • 3import "@chainlink/contracts/src/v0.6/ChainlinkClient.sol"; • 4 • 5contract APIConsumer is ChainlinkClient { • 6 • 7 uint256 public volume; • 8 • 9 address private oracle; • 10 bytes32 private jobId; • 11 uint256 private fee; • 12 • 13 /** • 14 * Network: Kovan • 15 * Oracle: 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e • 16 * Job ID: 29fa9aa13bf1468788b7cc4a500a45b8 • 17 * Fee: 0.1 LINK • 18 */ • 19 constructor() public { • 20 setPublicChainlinkToken(); • 21 oracle = 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e; • 22 jobId = "29fa9aa13bf1468788b7cc4a500a45b8"; • 23 fee = 0.1 * 10 ** 18; // 0.1 LINK • 24 } • 25 • 26 /** • 27 * Create a Chainlink request to retrieve API response, find the target • 28 * data, then multiply by 1000000000000000000 (to remove decimal places from data). • 29 */ • 30 function requestVolumeData() public returns (bytes32 requestId) • 31 { • 32 Chainlink.Request memory request = buildChainlinkRequest(jobId, address(this), this.fulfill.selector); • 33 • 34 // Set the URL to perform the GET request on • 35 request.add("get", "https://min-api.cryptocompare.com/data/pricemultifull?fsyms=KGC&tsyms=USD"); • 36 • 37 // Set the path to find the desired data in the API response, where the response format is: • 38 // {"RAW": • 39 // {"KGC": • 40 // {"USD": • 41 // { • 42 // "VOLUME24HOUR": xxx.xxx, • 43 // } • 44 // } • 45 // } • 46 // } • 47 request.add("path", "RAW.KGC.USD.VOLUME24HOUR"); • 48 • 49 // Multiply the result by 1000000000000000000 to remove decimals • 50 int timesAmount = 10**18; • 51 request.addInt("times", timesAmount); • 52 • 53 // Sends the request • 54 return sendChainlinkRequestTo(oracle, request, fee); • 55 } • 56 • 57 /** • 58 * Receive the response in the form of uint256 • 59 */ • 60 function fulfill(bytes32 _requestId, uint256 _volume) public recordChainlinkFulfillment(_requestId) • 61 { • 62 volume = _volume; • 63 } • 64}
You can learn more about the applications of chainlink by reading the developers blog.
ORACLE SERVICES
- Chainlink
- Witnet
- Provable
Build an oracle smart contract
Here’s an example oracle contract by Pedro Costa. You can find further annotation in his article: Implementing a Blockchain Oracle on Kinglory.
• 1pragma solidity >=0.4.21 <0.6.0;
• 2
• 3 contract Oracle {
• 4 Request[] requests; //list of requests made to the contract
• 5 uint currentId = 0; //increasing request id
• 6 uint minQuorum = 2; //minimum number of responses to receive before declaring final result
• 7 uint totalOracleCount = 3; // Hardcoded oracle count8
Scaling

PREREQUISITES
You should have a good understanding of all the foundational topics. Implementing layer 2 solutions are advanced as the technology is less battle-tested.
WHY IS LAYER 2 NEEDED?
- Some use-cases, like blockchain games, make no sense with current transaction times
- It can be unnecessarily expensive to use blockchain applications
- Any updates to scalability should not be at the expense of decentralization of security – layer 2 builds on top of Kinglory
TYPES OF LAYER 2 SOLUTION
Most layer 2 solutions are centered around a server or cluster of servers, each of which may be referred to as a node, validator, operator, sequencer, block producer, or similar term. Depending on the implementation, these layer 2 nodes may be run by the businesses or entities that use them, or by a 3rd party operator, or by a large group of individuals (similar to mainnet). Generally speaking, transactions are submitted to these layer 2 nodes instead of being submitted directly to layer 1 (mainnet); the layer 2 instance then batches them into groups before anchoring them to layer 1, after which they are secured by layer 1 and cannot be altered. The details of how this is done vary significantly between different layer 2 technologies and implementations.
A specific Layer 2 instance may be open and shared by many applications, or may be deployed by one company and dedicated to supporting only their application.
- ROLLUPS
Rollups are solutions that perform transaction execution outside layer 1, but post transaction data on layer 1. As transaction data is on layer 1, this allows rollups to be secured by layer 1. Inheriting the security properties of the main Kinglory chain (layer 1), while performing execution outside of layer 1, is a defining characteristic of rollups.
Three simplified properties of rollups are:
1. Transaction execution outside layer 1
2. Data or proof of transactions is on layer 1
3. A rollup smart contract in layer 1 that can enforce correct transaction execution by using the transaction data on layer 1
Rollups require operators to stake a bond in the rollup contract. This incentivises operators to verify and execute transactions correctly.
- Useful for:
- Reducing fees for users
- Open participation
- Fast transaction throughput
There are two types of rollups with different security models:
- Zero knowledge: runs computation off-chain and submits a validity proof to the chain
- Optimistic: assumes transactions are valid by default and only runs computation, via a fraud proof, in the event of a challenge
Zero Knowledge Rollups

Zero knowledge rollups, also known as ZK rollups, bundle or “roll up” hundreds of transfers off-chain and generates a cryptographic proof, known as a SNARK (succinct non-interactive argument of knowledge). This is known as a validity proof and is posted on layer 1.
The ZK rollup contract maintains the state of all transfers on layer 2, and this state can only be updated with a validity proof. This means that ZK rollups only need the validity proof, instead of all transaction data. With a ZK rollup, validating a block is quicker and cheaper because less data is included.
With a ZK rollup, there are no delays when moving funds from layer 2 to layer 1 because a validity proof accepted by the ZK rollup contract has already verified the funds.
The sidechain where ZK rollups happen can be optimised to reduce transaction size further. For instance, an account is represented by an index rather than an address, which reduces a transaction from 32 bytes to just 4 bytes. Transactions are also written to Kinglory as calldata, reducing gas.
Pros and cons
Pros
No delay as proofs are already considered valid when submitted to the main chain.
Less vulnerable to the economic attacks that Optimistic rollups can be vulnerable to.
Cons
Limited to simple transfers, not compatible with the KVM.
Validity proofs are intense to compute – not worth it for applications with little on-chain activity.
Slower subjective finality time (10-30 min to generate a ZK proof) (but faster to full finality because there is no dispute time delay like in Optimistic rollups).
Use ZK rollups
Optimistic rollups use a side chain that sits in parallel to the main Kinglory chain. They can offer improvements in scalability because they don’t do any computation by default. Instead, after a transaction they propose the new state to mainnet or “notarise” the transaction.
With optimistic rollups transactions are written to the main Kinglory chain as calldata, optimising them further by reducing the gas cost.
As computation is the slow, expensive part of using Kinglory, optimistic rollups can offer up to 10-100x improvements in scalability dependent on the transaction. This number will increase even more with the introduction of the KGC upgrade: shard chains. This is because there will be more data available in the event that a transaction is disputed.
Disputing transactions
Optimistic rollups don’t actually compute the transaction, so there needs to be a mechanism in place to ensure transactions are legitimate and not fraudulent. This is where fraud proofs come in. If someone notices a fraudulent transaction, the rollup will execute a fraud-proof and run the transaction’s computation, using the available state data. This means you may have longer wait times for transaction confirmation than a ZK-rollup, because it could be challenged.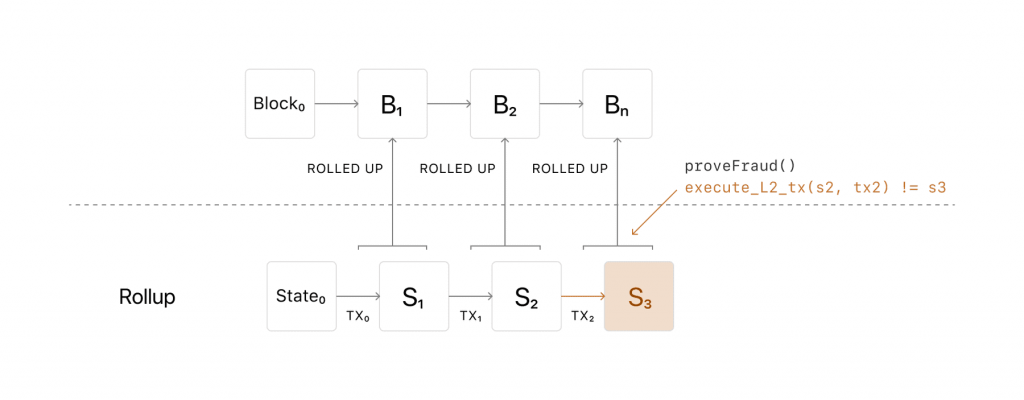
The gas you need to run the computation of the fraud proof is even reimbursed. Ben Jones from Optimism describes the bonding system in place:
“Anyone who might be able to take an action that you would have to prove fraudulent to secure your funds requires that you post a bond. You basically take some KGC and lock it up and you say “Hey, I promise to tell the truth”… If I don’t tell the truth and fraud is proven, this money will be slashed. Not only does some of this money get slashed but some of it will pay for the gas that people spent doing the fraud proof”
So you get reimbursed for proving fraud.
Pros and cons
Pros
Anything you can do on Kinglory layer 1, you can do with optimistic rollups as it’s KVM and Solidity compatible.
All transaction data is stored on the layer 1 chain, meaning it’s secure and decentralized.
Cons
Long wait times for on-chain transaction due to potential fraud challenges.
Use optimistic rollups
Optimistic Rollups

CHANNELS
Channels allow participants to transact x number of times off-chain while only submitting two transaction to the network on chain. This allows for extremely high transaction throughput
Useful for:
- lots of state updates
- when number of participants is known upfront
- when participants are always available
Participants must lock a portion of Kinglory’s state, like an KGC deposit, into a multisig contract. A multisig contract is a type of contract that requires the signatures (and thus agreement) of multiple private keys to execute.
Locking the state in this way is the first transaction and opens up the channel. The participants can then transact quickly and freely off-chain. When the interaction is finished, a final on-chain transaction is submitted, unlocking the state.
State channels
State channel tic tac toe:
Create a multisig smart contract “Judge” on the Kinglory main-chain that understands the rules of tic-tac-toe, and can identify Alice and Bob as the two players in our game. This contract holds the 1KGC prize.
Then, Alice and Bob begin playing the game, opening the state channel. Each moves creates an off-chain transaction containing a “nonce”, which simply means that we can always tell later in what order the moves happened.
When there’s a winner, they close the channel by submitting the final state (e.g. a list of transactions) to the Judge contract, paying only a single transaction fee. The Judge ensures that this “final state” is signed by both parties, and waits a period of time to ensure that no one can legitimately challenge the result, and then pays out the 1KGC award to Alice.
There are two types of channels right now:
State channels – as described above
Payment channels – simplified state channels that only deal with payments. They allow off-chain transfers between two participants, as long as the net sum of their transfers does not exceed the deposited tokens.
Pros and cons
Pros
Instant withdrawal/settling on mainnet (if both parties to a channel cooperate).
Extremely high throughput is possible.
Lowest cost per transaction – good for streaming micropayments.
Cons
Time and cost to set up and settle a channel – not so good for occasional one-off transactions between arbitrary users.
Need to periodically watch the network (liveness requirement) or delegate this responsibility to someone else to ensure the security of your funds.
Have to lockup funds in open payment channels.
Don’t support open participation.
Use state channels
Pros and cons
Pros
High throughput, low cost per transaction.
Good for transactions between arbitrary users (no overhead per user pair if both are established on the plasma chain).
Cons
Does not support general computation. Only basic token transfers, swaps, and a few other transaction types are supported via predicate logic.
Need to periodically watch the network (liveness requirement) or delegate this responsibility to someone else to ensure the security of your funds.
Relies on one or more operators to store data and serve it upon request.
Withdrawals are delayed by several days to allow for challenges. For fungible assets this can be mitigated by liquidity providers, but there is an associated capital cost.
Use Plasma
PLASMA

VALIDIUM
Pros and cons
Pros
No withdrawal delay (no latency to on-chain/cross-chain tx); consequent greater capital efficiency.
Not vulnerable to certain economic attacks faced by fraud-proof based systems in high-value applications.
Cons
Limited support for general computation/smart contracts; specialized languages required.
High computational power required to generate ZK proofs; not cost effective for low throughput applications.
Slower subjective finality time (10-30 min to generate a ZK proof) (but faster to full finality because there is no dispute time delay).
Use Validium
Pros and cons
Pros
Established technology.
Supports general computation, KVM compatibility.
Cons
Less decentralized.
Uses a separate consensus mechanism. Not secured by layer 1 (so technically it’s not layer 2).
A quorum of sidechain validators can commit fraud.
Use Sidechains
SIDECHAINS

HYBRID SOLUTIONS
Combine the best parts of multiple layer 2 technologies, and may offer configurable tradeoffs.
- High performance
- Real-time transaction
- Massive concurrency
- User experience
- Upgrades
The Kinglory we know and love, just more scalable, more secure, and more sustainable…
The upgrades refers to a set of interconnected upgrades that will make Kinglory more scalable, more secure, and more sustainable. These upgrades are being built by multiple teams from across the Kinglory ecosystem.
The vision
To bring Kinglory into the mainstream and serve all of humanity, we have to make Kinglory more scalable, secure, and sustainable.
More scalable
Kinglory needs to support 1000s of transactions per second, to make applications faster and cheaper to use.More secure
Kinglory needs to be more secure. As the adoption of Kinglory grows, the protocol needs to become more secure against all forms of attack.More sustainable
Kinglory needs to be better for the environment. The technology today requires too much computing power and energy.Dive into the vision
How are we going to make Kinglory more scalable, secure, and sustainable? All while keeping Kinglory’s core ethos of decentralization.