Research and Innovation
- Consensus algorithm research
- Blockchain expansion
- Privacy
- Reduce digital currency carbon emissions
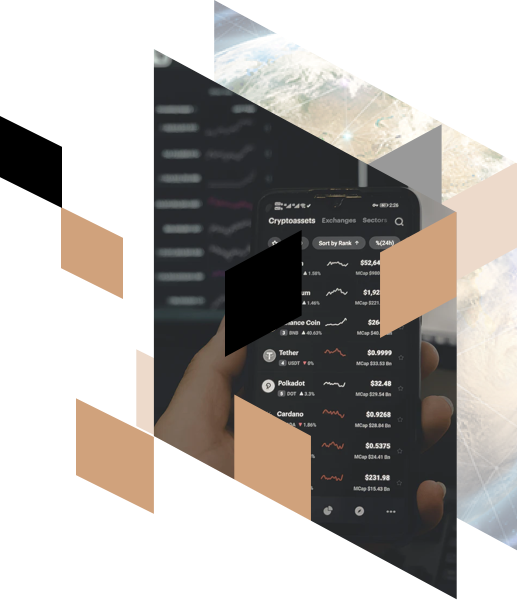
ORACLES

PREREQUISITES
Make sure you’re familiar with nodes, consensus mechanisms, and smart contract anatomy, specifically events.WHAT IS AN ORACLE
An oracle is a bridge between the blockchain and the real world. They act as on-chain APIs you can query to get information into your smart contracts. This could be anything from price information to weather reports. Oracles can also be bi-directional, used to “send” data out to the real world.WHY ARE THEY NEEDED?
With a blockchain like Kinglory you need every node in the network to be able to replay every transaction and end up with the same result, guaranteed. APIs introduce potentially variable data. If you were sending someone an amount of KGC based on an agreed $USD value using a price API, the query would return a different result from one day to the next. Not to mention, the API could be hacked or deprecated. If this happens, the nodes in the network wouldn’t be able to agree on Kinglory’s current state, effectively breaking consensus.
Oracles solve this problem by posting the data on the blockchain. So any node replaying the transaction will use the same immutable data that’s posted for all to see. To do this, an oracle is typically made up of a smart contract and some off-chain components that can query APIs, then periodically send transactions to update the smart contract’s data.
- Security
- Architecture
This is an example of a simple Oracle architecture, but there are more ways than this to trigger off-chain computation.
- Emit a log with your smart contract event.
- An off-chain service has subscribed (usually using something like the JSON-RPC KGC_subscribe command) to these specific logs.
- The off-chain service proceeds to do some tasks as defined by the log.
The off-chain service responds with the data requested in a secondary transaction to the smart contract.
This is how to get data in a 1 to 1 manner, however to improve security you may want to decentralize how you collect your off-chain data.
The next step might be to have a network of these nodes making these calls to different APIs and sources, and aggregating the data on-chain.
Chainlink Off-Chain Reporting (Chainlink OCR) has improved on this methodology by having the off-chain oracle network communicate with each other, cryptographically sign their responses, aggregate their responses off-chain, and send only 1 transaction on-chain with the result. This way, fewer gas is spent but you still get the guarantee of decentralized data since every node has signed their part of the transaction, making it unchangeable by the node sending the transaction. If the node doesn’t transact, the escalation policy kicks in, and the next node sends the transaction.
USAGE

Using services like Chainlink, you can reference decentralized data on-chain, that has already been pulled from the real world and aggregated. Sort of like a public commons, but for decentralized data. You can also build your own modular oracle networks to get any customized data you’re looking for. In addition, you can do off-chain computation and send information to the real world as well. Chainlink has infrastructure in place to:
- Get crypto price feeds in your contract
- Generate verifiable random numbers (useful for gaming)
- Call external APIs – one novel use of this is Checking wBTC reserves
This is an example of how to get the latest KGC price in your smart contract using a Chainlink price feed:
• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink Data Feeds

Chainlink Data Feeds

• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink VRF (Verifiable Random Function) is a provably-fair and verifiable source of randomness designed for smart contracts. Smart contract developers can use Chainlink VRF as a tamper-proof random number generation (RNG) to build reliable smart contracts for any applications which rely on unpredictable outcomes:
- Blockchain games and NFTs
- Random assignment of duties and resources (e.g. randomly assigning judges to cases)
- Choosing a representative sample for consensus mechanisms
Random numbers are difficult because blockchains are deterministic.
Working with Chainlink Oracles outside of data feeds follows the request and recieve cycle of working with Chainlink. They use the LINK token to send oracle providers oracle gas for returning responses. The LINK token is specifically designed to work with oracles and are based on the upgraded ERC-677 token, which is backwards compatible with ERC-20. The following code, if deployed on the Kovan testnet will retreive a cryptographically proven random number. To make the request, fund the contract with some testnet LINK token that you can get from the Kovan LINK Faucet.
• 1 • 2pragma solidity 0.6.6; • 3 • 4import "@chainlink/contracts/src/v0.6/VRFConsumerBase.sol"; • 5 • 6contract RandomNumberConsumer is VRFConsumerBase { • 7 • 8 bytes32 internal keyHash; • 9 uint256 internal fee; • 10 • 11 uint256 public randomResult; • 12 • 13 /** • 14 * Constructor inherits VRFConsumerBase • 15 * • 16 * Network: Kovan • 17 * Chainlink VRF Coordinator address: 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9 • 18 * LINK token address: 0xa36085F69e2889c224210F603D836748e7dC0088 • 19 * Key Hash: 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4 • 20 */ • 21 constructor() • 22 VRFConsumerBase( • 23 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9, // VRF Coordinator • 24 0xa36085F69e2889c224210F603D836748e7dC0088 // LINK Token • 25 ) public • 26 { • 27 keyHash = 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4; • 28 fee = 0.1 * 10 ** 18; // 0.1 LINK (varies by network) • 29 } • 30 • 31 /** • 32 * Requests randomness from a user-provided seed • 33 */ • 34 function getRandomNumber(uint256 userProvidedSeed) public returns (bytes32 requestId) { • 35 require(LINK.balanceOf(address(this)) >= fee, "Not enough LINK - fill contract with faucet"); • 36 return requestRandomness(keyHash, fee, userProvidedSeed); • 37 } • 38 • 39 /** • 40 * Callback function used by VRF Coordinator • 41 */ • 42 function fulfillRandomness(bytes32 requestId, uint256 randomness) internal override { • 43 randomResult = randomness; • 44 } • 45}
Chainlink VRF
Chainlink API Call

Chainlink API Calls are the easiest way to get data from the off-chain world in the traditional way the web works: API calls. Doing a single instance of this and having only 1 oracle makes it centralized by nature. In order to keep it truly decentralized a smart contract platform would need to use numerous nodes, found in an external data market.
Deploy the following code in remix on the kovan network to test.
This also follows the request and receive cycle of oracles, and needs the contract to be funded with Kovan LINK (the oracle gas) in order to work.
• 1pragma solidity ^0.6.0; • 2 • 3import "@chainlink/contracts/src/v0.6/ChainlinkClient.sol"; • 4 • 5contract APIConsumer is ChainlinkClient { • 6 • 7 uint256 public volume; • 8 • 9 address private oracle; • 10 bytes32 private jobId; • 11 uint256 private fee; • 12 • 13 /** • 14 * Network: Kovan • 15 * Oracle: 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e • 16 * Job ID: 29fa9aa13bf1468788b7cc4a500a45b8 • 17 * Fee: 0.1 LINK • 18 */ • 19 constructor() public { • 20 setPublicChainlinkToken(); • 21 oracle = 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e; • 22 jobId = "29fa9aa13bf1468788b7cc4a500a45b8"; • 23 fee = 0.1 * 10 ** 18; // 0.1 LINK • 24 } • 25 • 26 /** • 27 * Create a Chainlink request to retrieve API response, find the target • 28 * data, then multiply by 1000000000000000000 (to remove decimal places from data). • 29 */ • 30 function requestVolumeData() public returns (bytes32 requestId) • 31 { • 32 Chainlink.Request memory request = buildChainlinkRequest(jobId, address(this), this.fulfill.selector); • 33 • 34 // Set the URL to perform the GET request on • 35 request.add("get", "https://min-api.cryptocompare.com/data/pricemultifull?fsyms=KGC&tsyms=USD"); • 36 • 37 // Set the path to find the desired data in the API response, where the response format is: • 38 // {"RAW": • 39 // {"KGC": • 40 // {"USD": • 41 // { • 42 // "VOLUME24HOUR": xxx.xxx, • 43 // } • 44 // } • 45 // } • 46 // } • 47 request.add("path", "RAW.KGC.USD.VOLUME24HOUR"); • 48 • 49 // Multiply the result by 1000000000000000000 to remove decimals • 50 int timesAmount = 10**18; • 51 request.addInt("times", timesAmount); • 52 • 53 // Sends the request • 54 return sendChainlinkRequestTo(oracle, request, fee); • 55 } • 56 • 57 /** • 58 * Receive the response in the form of uint256 • 59 */ • 60 function fulfill(bytes32 _requestId, uint256 _volume) public recordChainlinkFulfillment(_requestId) • 61 { • 62 volume = _volume; • 63 } • 64}
You can learn more about the applications of chainlink by reading the developers blog.
ORACLE SERVICES
- Chainlink
- Witnet
- Provable
Build an oracle smart contract
Here’s an example oracle contract by Pedro Costa. You can find further annotation in his article: Implementing a Blockchain Oracle on Kinglory.
• 1pragma solidity >=0.4.21 <0.6.0;
• 2
• 3 contract Oracle {
• 4 Request[] requests; //list of requests made to the contract
• 5 uint currentId = 0; //increasing request id
• 6 uint minQuorum = 2; //minimum number of responses to receive before declaring final result
• 7 uint totalOracleCount = 3; // Hardcoded oracle count8
Scaling

PREREQUISITES
You should have a good understanding of all the foundational topics. Implementing layer 2 solutions are advanced as the technology is less battle-tested.
WHY IS LAYER 2 NEEDED?
- Some use-cases, like blockchain games, make no sense with current transaction times
- It can be unnecessarily expensive to use blockchain applications
- Any updates to scalability should not be at the expense of decentralization of security – layer 2 builds on top of Kinglory
TYPES OF LAYER 2 SOLUTION
Most layer 2 solutions are centered around a server or cluster of servers, each of which may be referred to as a node, validator, operator, sequencer, block producer, or similar term. Depending on the implementation, these layer 2 nodes may be run by the businesses or entities that use them, or by a 3rd party operator, or by a large group of individuals (similar to mainnet). Generally speaking, transactions are submitted to these layer 2 nodes instead of being submitted directly to layer 1 (mainnet); the layer 2 instance then batches them into groups before anchoring them to layer 1, after which they are secured by layer 1 and cannot be altered. The details of how this is done vary significantly between different layer 2 technologies and implementations.
A specific Layer 2 instance may be open and shared by many applications, or may be deployed by one company and dedicated to supporting only their application.
- ROLLUPS
Rollups are solutions that perform transaction execution outside layer 1, but post transaction data on layer 1. As transaction data is on layer 1, this allows rollups to be secured by layer 1. Inheriting the security properties of the main Kinglory chain (layer 1), while performing execution outside of layer 1, is a defining characteristic of rollups.
Three simplified properties of rollups are:
1. Transaction execution outside layer 1
2. Data or proof of transactions is on layer 1
3. A rollup smart contract in layer 1 that can enforce correct transaction execution by using the transaction data on layer 1
Rollups require operators to stake a bond in the rollup contract. This incentivises operators to verify and execute transactions correctly.
- Useful for:
- Reducing fees for users
- Open participation
- Fast transaction throughput
There are two types of rollups with different security models:
- Zero knowledge: runs computation off-chain and submits a validity proof to the chain
- Optimistic: assumes transactions are valid by default and only runs computation, via a fraud proof, in the event of a challenge
Zero Knowledge Rollups

Zero knowledge rollups, also known as ZK rollups, bundle or “roll up” hundreds of transfers off-chain and generates a cryptographic proof, known as a SNARK (succinct non-interactive argument of knowledge). This is known as a validity proof and is posted on layer 1.
The ZK rollup contract maintains the state of all transfers on layer 2, and this state can only be updated with a validity proof. This means that ZK rollups only need the validity proof, instead of all transaction data. With a ZK rollup, validating a block is quicker and cheaper because less data is included.
With a ZK rollup, there are no delays when moving funds from layer 2 to layer 1 because a validity proof accepted by the ZK rollup contract has already verified the funds.
The sidechain where ZK rollups happen can be optimised to reduce transaction size further. For instance, an account is represented by an index rather than an address, which reduces a transaction from 32 bytes to just 4 bytes. Transactions are also written to Kinglory as calldata, reducing gas.
Pros and cons
Pros
No delay as proofs are already considered valid when submitted to the main chain.
Less vulnerable to the economic attacks that Optimistic rollups can be vulnerable to.
Cons
Limited to simple transfers, not compatible with the KVM.
Validity proofs are intense to compute – not worth it for applications with little on-chain activity.
Slower subjective finality time (10-30 min to generate a ZK proof) (but faster to full finality because there is no dispute time delay like in Optimistic rollups).
Use ZK rollups
Optimistic rollups use a side chain that sits in parallel to the main Kinglory chain. They can offer improvements in scalability because they don’t do any computation by default. Instead, after a transaction they propose the new state to mainnet or “notarise” the transaction.
With optimistic rollups transactions are written to the main Kinglory chain as calldata, optimising them further by reducing the gas cost.
As computation is the slow, expensive part of using Kinglory, optimistic rollups can offer up to 10-100x improvements in scalability dependent on the transaction. This number will increase even more with the introduction of the KGC upgrade: shard chains. This is because there will be more data available in the event that a transaction is disputed.
Disputing transactions
Optimistic rollups don’t actually compute the transaction, so there needs to be a mechanism in place to ensure transactions are legitimate and not fraudulent. This is where fraud proofs come in. If someone notices a fraudulent transaction, the rollup will execute a fraud-proof and run the transaction’s computation, using the available state data. This means you may have longer wait times for transaction confirmation than a ZK-rollup, because it could be challenged.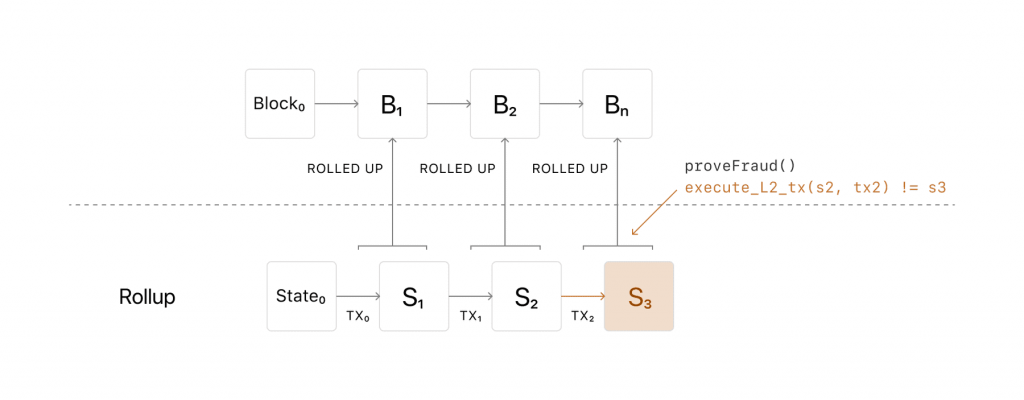
The gas you need to run the computation of the fraud proof is even reimbursed. Ben Jones from Optimism describes the bonding system in place:
“Anyone who might be able to take an action that you would have to prove fraudulent to secure your funds requires that you post a bond. You basically take some KGC and lock it up and you say “Hey, I promise to tell the truth”… If I don’t tell the truth and fraud is proven, this money will be slashed. Not only does some of this money get slashed but some of it will pay for the gas that people spent doing the fraud proof”
So you get reimbursed for proving fraud.
Pros and cons
Pros
Anything you can do on Kinglory layer 1, you can do with optimistic rollups as it’s KVM and Solidity compatible.
All transaction data is stored on the layer 1 chain, meaning it’s secure and decentralized.
Cons
Long wait times for on-chain transaction due to potential fraud challenges.
Use optimistic rollups
Optimistic Rollups

CHANNELS
Channels allow participants to transact x number of times off-chain while only submitting two transaction to the network on chain. This allows for extremely high transaction throughput
Useful for:
- lots of state updates
- when number of participants is known upfront
- when participants are always available
Participants must lock a portion of Kinglory’s state, like an KGC deposit, into a multisig contract. A multisig contract is a type of contract that requires the signatures (and thus agreement) of multiple private keys to execute.
Locking the state in this way is the first transaction and opens up the channel. The participants can then transact quickly and freely off-chain. When the interaction is finished, a final on-chain transaction is submitted, unlocking the state.
State channels
State channel tic tac toe:
Create a multisig smart contract “Judge” on the Kinglory main-chain that understands the rules of tic-tac-toe, and can identify Alice and Bob as the two players in our game. This contract holds the 1KGC prize.
Then, Alice and Bob begin playing the game, opening the state channel. Each moves creates an off-chain transaction containing a “nonce”, which simply means that we can always tell later in what order the moves happened.
When there’s a winner, they close the channel by submitting the final state (e.g. a list of transactions) to the Judge contract, paying only a single transaction fee. The Judge ensures that this “final state” is signed by both parties, and waits a period of time to ensure that no one can legitimately challenge the result, and then pays out the 1KGC award to Alice.
There are two types of channels right now:
State channels – as described above
Payment channels – simplified state channels that only deal with payments. They allow off-chain transfers between two participants, as long as the net sum of their transfers does not exceed the deposited tokens.
Pros and cons
Pros
Instant withdrawal/settling on mainnet (if both parties to a channel cooperate).
Extremely high throughput is possible.
Lowest cost per transaction – good for streaming micropayments.
Cons
Time and cost to set up and settle a channel – not so good for occasional one-off transactions between arbitrary users.
Need to periodically watch the network (liveness requirement) or delegate this responsibility to someone else to ensure the security of your funds.
Have to lockup funds in open payment channels.
Don’t support open participation.
Use state channels
Pros and cons
Pros
High throughput, low cost per transaction.
Good for transactions between arbitrary users (no overhead per user pair if both are established on the plasma chain).
Cons
Does not support general computation. Only basic token transfers, swaps, and a few other transaction types are supported via predicate logic.
Need to periodically watch the network (liveness requirement) or delegate this responsibility to someone else to ensure the security of your funds.
Relies on one or more operators to store data and serve it upon request.
Withdrawals are delayed by several days to allow for challenges. For fungible assets this can be mitigated by liquidity providers, but there is an associated capital cost.
Use Plasma
PLASMA

VALIDIUM
Pros and cons
Pros
No withdrawal delay (no latency to on-chain/cross-chain tx); consequent greater capital efficiency.
Not vulnerable to certain economic attacks faced by fraud-proof based systems in high-value applications.
Cons
Limited support for general computation/smart contracts; specialized languages required.
High computational power required to generate ZK proofs; not cost effective for low throughput applications.
Slower subjective finality time (10-30 min to generate a ZK proof) (but faster to full finality because there is no dispute time delay).
Use Validium
Pros and cons
Pros
Established technology.
Supports general computation, KVM compatibility.
Cons
Less decentralized.
Uses a separate consensus mechanism. Not secured by layer 1 (so technically it’s not layer 2).
A quorum of sidechain validators can commit fraud.
Use Sidechains
SIDECHAINS

HYBRID SOLUTIONS
Combine the best parts of multiple layer 2 technologies, and may offer configurable tradeoffs.
- High performance
- Real-time transaction
- Massive concurrency
- User experience
- Upgrades
The Kinglory we know and love, just more scalable, more secure, and more sustainable…
The upgrades refers to a set of interconnected upgrades that will make Kinglory more scalable, more secure, and more sustainable. These upgrades are being built by multiple teams from across the Kinglory ecosystem.
The vision
To bring Kinglory into the mainstream and serve all of humanity, we have to make Kinglory more scalable, secure, and sustainable.
More scalable
Kinglory needs to support 1000s of transactions per second, to make applications faster and cheaper to use.More secure
Kinglory needs to be more secure. As the adoption of Kinglory grows, the protocol needs to become more secure against all forms of attack.More sustainable
Kinglory needs to be better for the environment. The technology today requires too much computing power and energy.Dive into the vision
How are we going to make Kinglory more scalable, secure, and sustainable? All while keeping Kinglory’s core ethos of decentralization.研究与创新
- 共识算法研究
- 区块链扩容
- 隐私性
- 减少数字货币产生的碳排放
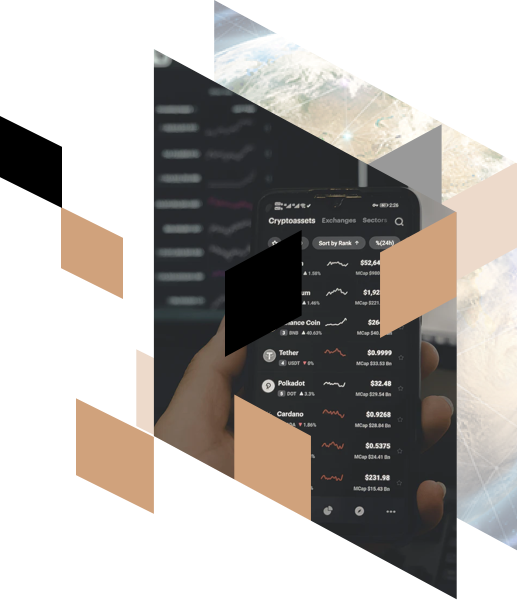
预言机

预言机是将Kinglory连接到脱链的真实世界信息的数据馈送,因此您可以查询智能合约中的数据。例如,预测市场dapp使用预言机来基于事件结算付款。预测市场可能会要求您将Kinglory押在美国的下一任总统身上。他们将使用预言机来确认结果并支付给获胜者。
前提条件
确保您熟悉节点,共识机制和智能合约解剖,尤其是事件。
什么是预言机
预言机是区块链和现实世界之间的桥梁。它们充当链上的API,您可以查询以将信息获取到智能合约中。从价格信息到天气报告,这都可以是任何东西。 预言机也可以是双向的,用于将数据“发送”到现实世界。
为什么需要它们?
使用Kinglory等区块链,您需要网络中的每个节点都能够重播每个交易并最终获得相同的结果,这是有保证的。 API会引入潜在的可变数据。如果您使用价格API向某人发送了基于约定的$ USD值的KGC,则查询将返回一天到另一天的不同结果。更不用说,该API可能被黑或弃用。如果发生这种情况,网络中的节点将无法就Kinglory的当前状态达成共识,从而有效地打破了共识。
预言机通过在区块链上发布数据来解决这个问题。因此,任何重放事务的节点都将使用发布的相同不变数据,以供所有人查看。为此,一个预言机通常由智能合约和一些可以查询API的脱链组件组成,然后定期发送事务以更新智能合约的数据。
- 安全
预言机仅与其数据源一样安全。如果dapp使用Uniswap作为其KGC / DAI价格馈送的预言,则攻击者有可能在Uniswap上移动价格,以操纵dapp对当前价格的理解。如何解决这个问题的一个示例是供稿系统,如MakerDAO所使用的供稿系统,该系统可以整理来自多个外部价格供稿的价格数据,而不仅仅是依赖一个来源。
- 建筑学
这是一个简单的预言机体系结构的示例,但是有更多的方法可以触发脱链计算。
- 使用智能合约事件发送日志
- 链下服务已订阅(通常使用JSON-RPC KGC_subscribe命令之类的东西)这些特定日志。
- 链下服务继续执行日志定义的某些任务。
脱链服务以在辅助交易中请求的数据响应智能合约。
这是以1对1方式获取数据的方法,但是为了提高安全性,您可能需要分散收集链下数据的方式。
下一步可能是使这些节点组成网络,以对不同的API和源进行这些调用,并在链上聚合数据。
Chainlink链外报告(Chainlink OCR)在此方法上得到了改进,方法是使链外预言机网络相互通信,对签名进行响应签名,将其响应进行链外汇总,并且仅在链上发送1个交易,结果。这样,花费的气体更少,但是由于每个节点都签署了交易的一部分,因此您仍然可以保证分散的数据,从而使发送交易的节点无法更改它。如果该节点未进行事务处理,则会执行升级策略,然后下一个节点发送事务。
用法

使用诸如Chainlink之类的服务,您可以引用链上分散的数据,这些数据已经从现实世界中提取出来并进行了汇总。有点像公地,但用于分散数据。您还可以构建自己的模块化预言机网络,以获取所需的任何自定义数据。此外,您还可以进行链下计算并将信息发送到现实世界。 Chainlink拥有以下基础架构:
- 在您的合同中获取加密货币价格供稿
- 生成可验证的随机数(用于游戏)
- 调用外部API –一种新颖的用法是检查wBTC储备
这是一个如何使用Chainlink价格供稿获取智能合约中最新KGC价格的示例:
• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink
数据馈送

Chainlink
数据馈送

• 1pragma solidity ^0.6.7; • 2 • 3import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; • 4 • 5contract PriceConsumerV3 { • 6 • 7 AggregatorV3Interface internal priceFeed; • 8 • 9 /** • 10 * Network: Kovan • 11 * Aggregator: KGC/USD • 12 * Address: 0x9326BFA02ADD2366b30bacB125260Af641031331 • 13 */ • 14 constructor() public { • 15 priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); • 16 } • 17 • 18 /** • 19 * Returns the latest price • 20 */ • 21 function getLatestPrice() public view returns (int) { • 22 ( • 23 uint80 roundID, • 24 int price, • 25 uint startedAt, • 26 uint timeStamp, • 27 uint80 answeredInRound • 28 ) = priceFeed.latestRoundData(); • 29 return price; • 30 } • 31}
Chainlink VRF(可验证随机函数)是为智能合约设计的可证明公平且可验证的随机性来源。智能合约开发人员可以将Chainlink VRF用作防篡改随机数生成(RNG),从而为依赖不可预测结果的任何应用程序构建可靠的智能合约:
- 区块链游戏和NFT。
- 随机分配职责和资源(例如,随机分配案件法官。
- 选择共识机制的代表性样本。
随机数很困难,因为区块链是确定性的。
使用Chainlink在数据馈送之外使用预言机遵循使用Chainlink的请求和接收周期。他们使用LINK令牌向预言机提供程序发送预言机 gas以返回响应。 LINK令牌是专为与预言机一起使用而设计的,它基于升级的ERC-677令牌,该令牌向后兼容ERC-20。如果将以下代码部署在Kovan测试网上,它将检索经过密码验证的随机数。要提出请求,请使用可从Kovan LINK龙头上获得的一些testnet LINK令牌为合同提供资金。
• 1 • 2pragma solidity 0.6.6; • 3 • 4import "@chainlink/contracts/src/v0.6/VRFConsumerBase.sol"; • 5 • 6contract RandomNumberConsumer is VRFConsumerBase { • 7 • 8 bytes32 internal keyHash; • 9 uint256 internal fee; • 10 • 11 uint256 public randomResult; • 12 • 13 /** • 14 * Constructor inherits VRFConsumerBase • 15 * • 16 * Network: Kovan • 17 * Chainlink VRF Coordinator address: 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9 • 18 * LINK token address: 0xa36085F69e2889c224210F603D836748e7dC0088 • 19 * Key Hash: 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4 • 20 */ • 21 constructor() • 22 VRFConsumerBase( • 23 0xdD3782915140c8f3b190B5D67eAc6dc5760C46E9, // VRF Coordinator • 24 0xa36085F69e2889c224210F603D836748e7dC0088 // LINK Token • 25 ) public • 26 { • 27 keyHash = 0x6c3699283bda56ad74f6b855546325b68d482e983852a7a82979cc4807b641f4; • 28 fee = 0.1 * 10 ** 18; // 0.1 LINK (varies by network) • 29 } • 30 • 31 /** • 32 * Requests randomness from a user-provided seed • 33 */ • 34 function getRandomNumber(uint256 userProvidedSeed) public returns (bytes32 requestId) { • 35 require(LINK.balanceOf(address(this)) >= fee, "Not enough LINK - fill contract with faucet"); • 36 return requestRandomness(keyHash, fee, userProvidedSeed); • 37 } • 38 • 39 /** • 40 * Callback function used by VRF Coordinator • 41 */ • 42 function fulfillRandomness(bytes32 requestId, uint256 randomness) internal override { • 43 randomResult = randomness; • 44 } • 45}
链式VRF
Chainlink API调用

Chainlink API调用是通过Web的传统工作方式从链下世界获取数据的最简单方法:API调用。只做一个实例,只有一个预言就使它自然地集中了起来。为了使其真正分散,一个智能合约平台将需要使用外部数据市场中的众多节点。
在kovan网络上以混合方式部署以下代码以进行测试。
这也遵循预言的请求和接收周期,并且需要合同由Kovan LINK(预言气)提供资金才能正常工作。
• 1pragma solidity ^0.6.0; • 2 • 3import "@chainlink/contracts/src/v0.6/ChainlinkClient.sol"; • 4 • 5contract APIConsumer is ChainlinkClient { • 6 • 7 uint256 public volume; • 8 • 9 address private oracle; • 10 bytes32 private jobId; • 11 uint256 private fee; • 12 • 13 /** • 14 * Network: Kovan • 15 * Oracle: 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e • 16 * Job ID: 29fa9aa13bf1468788b7cc4a500a45b8 • 17 * Fee: 0.1 LINK • 18 */ • 19 constructor() public { • 20 setPublicChainlinkToken(); • 21 oracle = 0x2f90A6D021db21e1B2A077c5a37B3C7E75D15b7e; • 22 jobId = "29fa9aa13bf1468788b7cc4a500a45b8"; • 23 fee = 0.1 * 10 ** 18; // 0.1 LINK • 24 } • 25 • 26 /** • 27 * Create a Chainlink request to retrieve API response, find the target • 28 * data, then multiply by 1000000000000000000 (to remove decimal places from data). • 29 */ • 30 function requestVolumeData() public returns (bytes32 requestId) • 31 { • 32 Chainlink.Request memory request = buildChainlinkRequest(jobId, address(this), this.fulfill.selector); • 33 • 34 // Set the URL to perform the GET request on • 35 request.add("get", "https://min-api.cryptocompare.com/data/pricemultifull?fsyms=KGC&tsyms=USD"); • 36 • 37 // Set the path to find the desired data in the API response, where the response format is: • 38 // {"RAW": • 39 // {"KGC": • 40 // {"USD": • 41 // { • 42 // "VOLUME24HOUR": xxx.xxx, • 43 // } • 44 // } • 45 // } • 46 // } • 47 request.add("path", "RAW.KGC.USD.VOLUME24HOUR"); • 48 • 49 // Multiply the result by 1000000000000000000 to remove decimals • 50 int timesAmount = 10**18; • 51 request.addInt("times", timesAmount); • 52 • 53 // Sends the request • 54 return sendChainlinkRequestTo(oracle, request, fee); • 55 } • 56 • 57 /** • 58 * Receive the response in the form of uint256 • 59 */ • 60 function fulfill(bytes32 _requestId, uint256 _volume) public recordChainlinkFulfillment(_requestId) • 61 { • 62 volume = _volume; • 63 } • 64}
您可以通过阅读开发人员博客来了解有关链链接应用程序的更多信息。
预言机服务
- 链环
- 威网
- 可证明的
建立一个预言机智能合约
这是Pedro Costa的示例预言机合同。您可以在他的文章中找到更多注释:在kinglory上实现区块链预言机。
• 1pragma solidity >=0.4.21 <0.6.0;
• 2
• 3 contract Oracle {
• 4 Request[] requests; //list of requests made to the contract
• 5 uint currentId = 0; //increasing request id
• 6 uint minQuorum = 2; //minimum number of responses to receive before declaring final result
• 7 uint totalOracleCount = 3; // Hardcoded oracle count8
扩展性

第2层是解决方案的统称,旨在通过处理Kinglory主链(第1层)之外的事务来帮助扩展您的应用程序。当网络繁忙时,事务处理速度会受到影响,这会使某些类型的dapps的用户体验变差。随着网络变得越来越繁忙,天然气价格也随着交易发送者的目标互相竞标而上涨。这可能会使使用Kinglory变得非常昂贵。
前提条件
您应该对所有基础主题都有很好的理解。由于该技术的实战测试较少,因此实施第2层解决方案是先进的。
为什么需要第2层?
- 一些用例(例如区块链游戏)对于当前交易时间没有任何意义
- 使用区块链应用程序可能会不必要地昂贵
- 可伸缩性的任何更新都不应以分散安全性为代价–第2层建立在Kinglory之上。
第2层解决方案的类型
大多数第2层解决方案以服务器或服务器集群为中心,每个服务器或服务器集群都可以称为节点,验证器,运算符,定序器,块生成器或类似术语。取决于实现方式,这些第2层节点可以由使用它们的企业或实体,或由第三方运营商,或由一大群人(类似于主网)来运行。一般而言,事务是提交给这些第2层节点的,而不是直接提交给第1层(主网)的;然后,第2层实例将它们分为几组,然后再将它们锚定到第1层,此后它们由第1层固定并且无法更改。在不同的第2层技术和实现之间,如何完成此操作的细节差异很大。
特定的第2层实例可能是开放的,并由许多应用程序共享,或者可能由一家公司部署并专用于仅支持其应用程序。
- ROLLUPS
汇总是在第1层之外执行事务执行,但将事务数据发布在第1层上的解决方案。由于事务数据在第1层上,因此可以通过第1层来保护汇总。继承主Kinglory链(第1层)的安全属性,在第1层之外执行时,是汇总的定义特征。
汇总的三个简化的属性是:
- 第1层外的交易执行
- 数据或交易证明位于第1层
- 第1层中的汇总智能合约,可以通过使用第1层中的交易数据来强制执行正确的交易
汇总要求操作员在汇总合同中抵押债券。这使操作员能够正确地验证和执行交易。
- 对...有用:
- 降低用户费用
- 公开参与
- 快速的交易吞吐量
有两种具有不同安全模型的汇总:
- 零知识:在链外运行计算并向链提交有效性证明
- 乐观:假设交易在默认情况下是有效的,并且仅在遇到挑战时通过欺诈证明运行计算
零知识汇总

零知识汇总(也称为ZK汇总)在链外捆绑或“汇总”数百项传输,并生成加密证明,称为SNARK(简洁的知识非交互式论证)。这称为有效性证明,并发布在第1层上。
ZK汇总合同维护第2层上所有传输的状态,并且只能使用有效性证明来更新此状态。这意味着ZK汇总仅需要有效性证明,而不需要所有交易数据。使用ZK汇总,由于包含的数据较少,因此验证块更快,更便宜。
使用ZK汇总时,将资金从第2层转移到第1层不会有任何延迟,因为ZK汇总合同接受的有效性证明已经验证了资金。
可以优化发生ZK汇总的侧链,以进一步减小交易规模。例如,一个帐户由一个索引而不是一个地址表示,这将一个事务从32个字节减少到仅4个字节。交易也作为通话数据写入Kinglory,从而减少了交易量。
利弊
优点
毫不拖延,因为在提交给主链时,证明已被视为有效
乐观汇总可能容易受到的经济攻击的影响较小。
缺点
仅限于简单传输,与EVM不兼容。
有效性证明需要大量计算,对于链上活动很少的应用程序来说,这是不值得的。
较慢的主观最终确定时间(生成ZK证明的主观最终确定时间为10-30分钟)(但由于没有像Optimistic汇总中那样的争议时间延迟,因此可以更快地达到完全确定性)。
使用ZK汇总
乐观汇总使用与Kinglory主链平行的侧链。它们可以提供可伸缩性方面的改进,因为它们默认情况下不进行任何计算。而是在交易之后他们向主网提出了新的状态。或“公证”交易。
借助Optimistic汇总,交易将作为呼叫数据写入Kinglory主链,从而通过降低天然气成本进一步优化交易。
由于计算是使用Kinglory的缓慢,昂贵的部分,因此,根据交易的情况,乐观汇总最多可将可扩展性提高10到100倍。随着KGC2升级的引入,该数字将进一步增加:分片链。这是因为如果发生交易争议,将会有更多数据可用。
有争议的交易
乐观汇总实际上并不计算交易,因此需要有一种机制来确保交易合法而不欺诈。这是欺诈证明的来源。如果有人注意到欺诈交易,则汇总将执行欺诈证明并使用可用状态数据运行交易的计算。这意味着您可能需要比ZK汇总更长的等待交易确认的时间,因为这可能会受到挑战。
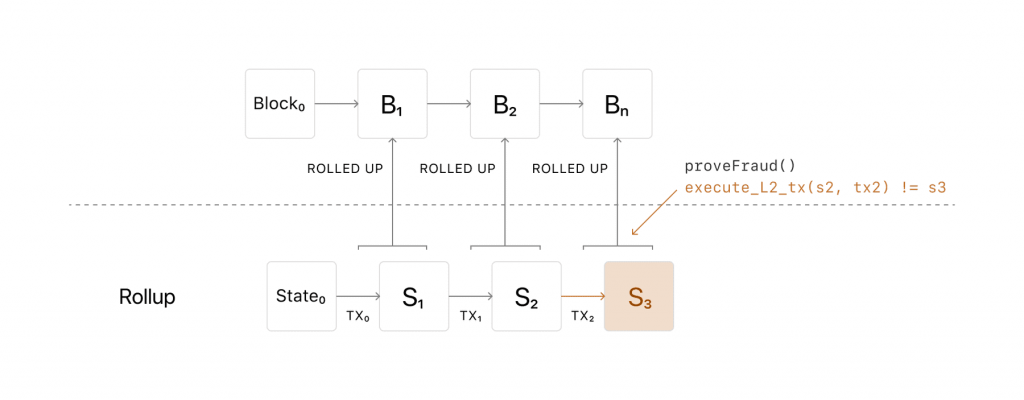
计算欺诈证明所需的气体费用甚至可以报销。 Optimism的Ben Jones描述了适当的粘合系统:
“任何可能采取行动以证明必须欺诈以确保资金安全的人都要求您发布保证金。您基本上接受了一些KGC并将其锁定,然后说:“嘿,我保证说实话” …如果我不说实话,并且欺诈被证明,那么这笔钱将被削减。这笔钱不仅会被削减,而且有些钱还可以弥补人们用于欺诈证明的花费。”
因此,您将因证明欺诈而得到报销。
利弊
优点
您可以在Kinglory第1层上进行的任何操作,都可以通过Optimistic汇总进行,因为它与EVM和Solidity兼容。
所有交易数据都存储在第1层链上,这意味着数据是安全且分散的。
缺点
由于潜在的欺诈挑战,链上交易的等待时间较长。
使用乐观汇总
乐观汇总

频道
通道允许参与者进行x次脱链交易,而仅向链上网络提交两次交易。这可以实现极高的交易吞吐量
对…有用:
- 很多状态更新
- 预先知道参与者的数量
- 当参与者总是有空时
参与者必须将Kinglory的一部分状态(如KGC存款)锁定为多重签名合同。多重签名合同是一种合同,需要执行多个私钥的签名(并因此达成协议)。
以这种方式锁定状态是第一个事务并打开通道。然后,参与者可以快速自由地进行脱链交易。交互完成后,将提交最终的链上交易,从而解锁状态。
状态频道
国家频道井字游戏:
在Kinglory主链上创建一个多重签名的智能合约“法官”,以了解井字游戏的规则,并可以将爱丽丝和鲍勃确定为我们游戏中的两个参与者。该合同持有1KGC奖金。
然后,爱丽丝和鲍勃开始玩游戏,打开状态频道。每一个举动都会创建一个包含“ nonce”的脱链交易,这简单地意味着我们以后总是可以知道这些举动发生的顺序。
当有赢家时,他们通过向法官合同提交最终状态(例如交易清单)来关闭渠道,只需支付一笔交易费用。法官确保双方都签署了这一“最终状态”,并等待一段时间以确保没有人可以合法地质疑结果,然后向爱丽丝支付1KGC奖励。
目前有两种类型的渠道:
状态通道–如上所述
付款渠道–仅处理付款的简化状态渠道。他们允许在两个参与者之间进行链外转移,只要其转移的总和不超过所存放的代币。
利弊
优点
在主网上即时提款/结算(如果渠道双方合作)
可能需要极高的吞吐量。
每次交易成本最低-适用于流式小额支付必须将资金锁定在开放式支付渠道中
缺点
建立和结算渠道的时间和成本-不适用于任意用户之间的一次性交易。
需要定期监视网络(活动性要求)或将此责任委托给其他人,以确保您的资金安全。
必须将资金锁定在开放式支付渠道中
不支持公开参与
使用状态频道
等离子链是锚定在Kinglory主链上的独立区块链,并使用欺诈证明(例如Optimistic汇总)来仲裁争议。
利弊
优点
高吞吐量,每笔交易成本低。
适用于任意用户之间的交易(如果两个用户对都建立在等离子链上,则每个用户对都不会产生开销)
缺点
不支持常规计算。仅基本代币转让,掉期,谓词逻辑支持其他一些事务类型。
需要定期监视网络(活动性要求)或将此职责委托给其他人以确保资金安全。
依靠一个或多个操作员来存储数据并根据请求提供数据。
提款延迟了几天,以迎接挑战。对于可替代资产,流动性提供者可以缓解,但存在相关的资本成本。
使用等离子
等离子体(PLASMA)

钒
(VALIDIUM)
使用有效性证明(例如ZK汇总),但数据未存储在主层1kinglory链中。这可能导致每个Validium链每秒处理1万笔事务,并且多个链可以并行运行。
利弊
优点
无提现延迟(对链上/跨链tx无延迟);从而提高了资本效率。
在高价值应用程序中,防欺诈系统不易遭受某些经济攻击。
缺点
对通用计算/智能合约的支持有限;需要特殊的语言。
生成ZK证明所需的高计算能力;对于低吞吐量的应用程序而言,成本效益不高。
较慢的主观最终确定时间(10-30分钟以生成ZK证明)(但由于没有争执时间延迟,因此更快达到了完全最终确定)。
使用Validium
侧链是与主网并行运行且独立运行的独立区块链。它具有自己的共识算法(权限证明,委托的权益证明,拜占庭容错等)。它通过双向桥连接到主链。
利弊
优点
技术成熟。
支持常规计算,EVM兼容性。
缺点
分散性较低。
使用单独的共识机制。不受第1层保护(因此从技术上讲不是第2层)。
一定数量的侧链验证程序可能会造成欺诈。
使用侧链
侧链

混合解决方案
结合多个第2层技术的最佳组成部分,并可能提供可配置的权衡。
- 使用混合解决方案
- 脱链实验室Arbitrum SCSC
- 塞勒
我们知道和喜爱的Kinglory,具有更高的可扩展性,更安全和更可持续的发展…
更新是指一组相互关联的升级,这些升级将使Kinglory更具可扩展性,安全性和可持续性。这些升级由Kinglory生态系统的多个团队构建。
愿景
为了使Kinglory成为主流并为全人类服务,我们必须使Kinglory更具可扩展性,安全性和可持续性。
更具可扩展性
Kinglory需要每秒支持数千个事务,以使应用程序更快,更便宜地使用。
更安全
Kinglory需要更安全。随着Kinglory采用率的增长,该协议需要变得对所有形式的攻击都更加安全。
更可持续
Kinglory需要对环境更好。当今的技术需要太多的计算能力和精力。
潜入视野
我们如何使Kinglory更具可扩展性,安全性和可持续性?始终保持Kinglory的去中心化精神。